File upload with Html5 & WebApi &
SignalR (3)
1. Web API with async upload
method.
2. Add SignalR framework to the Web API.
3.
Implement the front-end.
1. We will use MVC + Html5 to conduct the
front-end. My page was designed like the following picture.
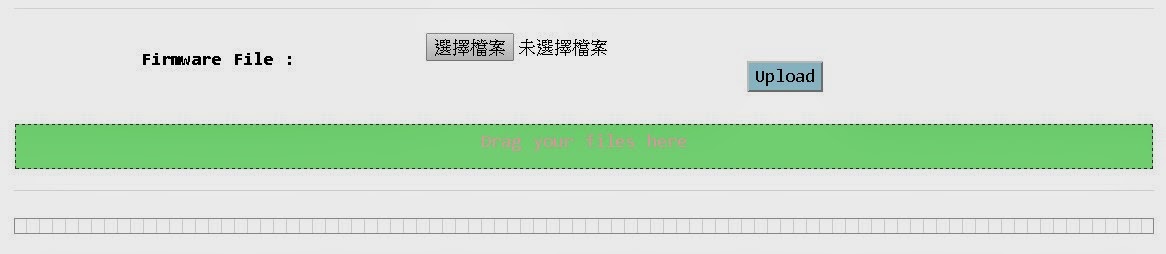
In this page, we are able to upload files by dragging multi files into the div block or using the fileBrowser html component.
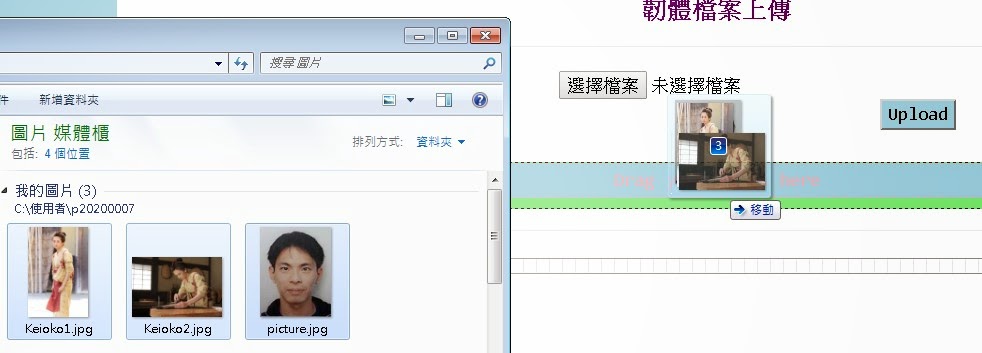
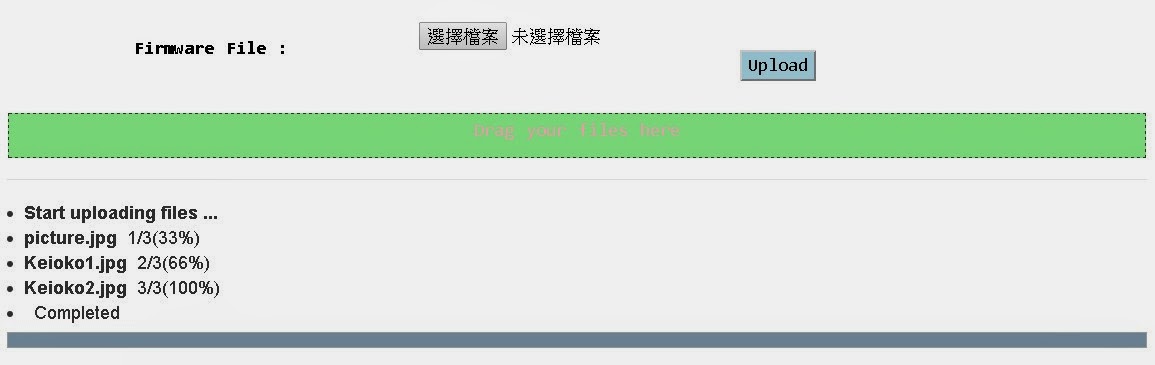
Files uploaded successfully.
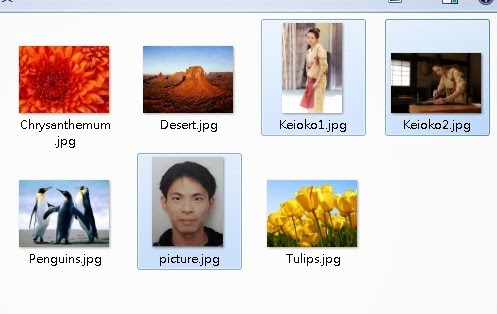
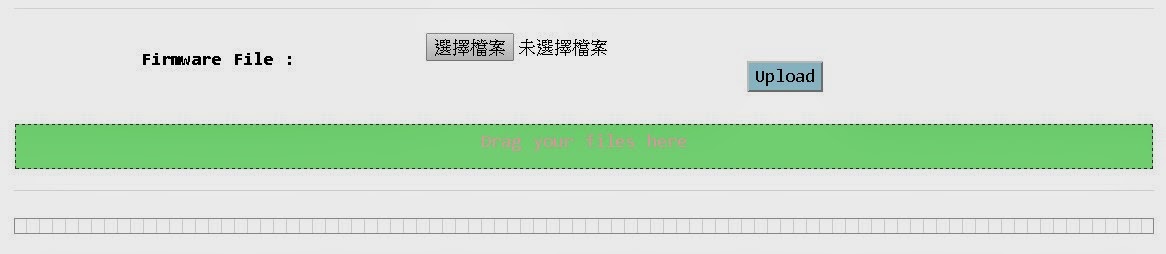
In this page, we are able to upload files by dragging multi files into the div block or using the fileBrowser html component.
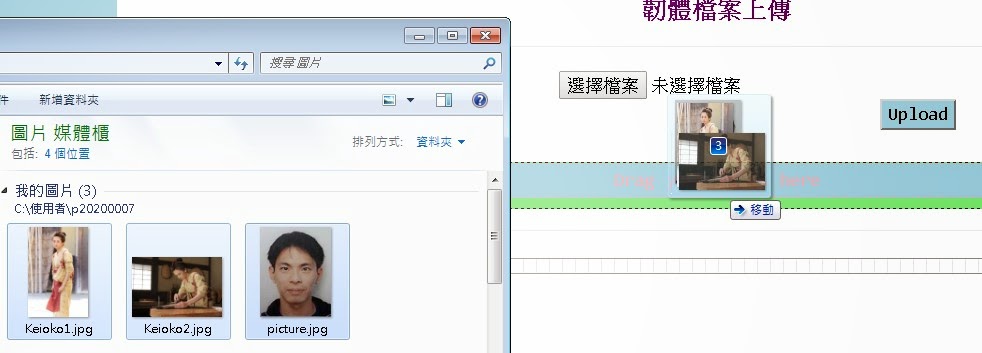
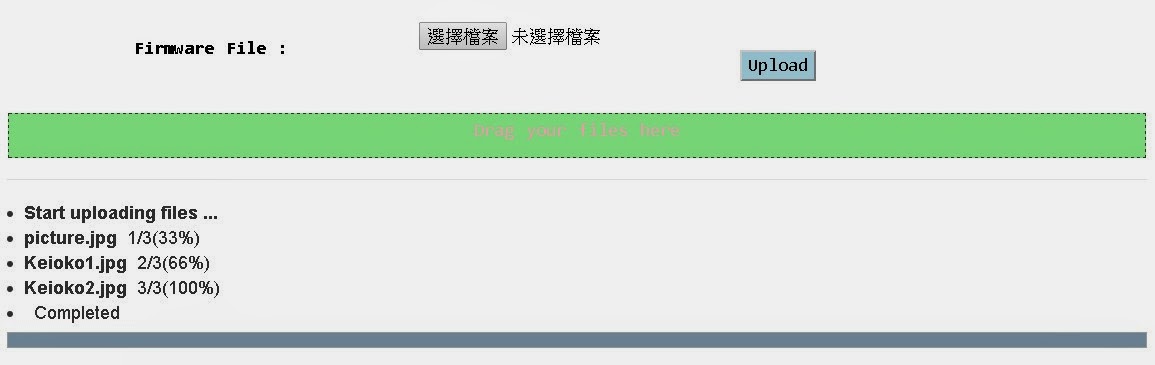
Files uploaded successfully.
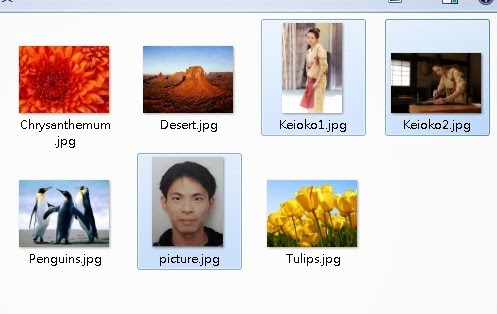
2. Let’s begin the implement. First, we
should import the SignalR architecture to our website as well.
3. View
The most important thing is that we must include these scripts :
■ SignalR js
■ The script created from the SignalR’s hub :
In this case, the script created from the SignalR’s hub is directed to WebAPI, so the url is http://[WebAPI host url]/SignalR/js
If u have the SignalR hub in this website, the script’s url is “/SignalR/js”.
The most important thing is that we must include these scripts :
■ SignalR js
■ The script created from the SignalR’s hub :
In this case, the script created from the SignalR’s hub is directed to WebAPI, so the url is http://[WebAPI host url]/SignalR/js
If u have the SignalR hub in this website, the script’s url is “/SignalR/js”.
@{
ViewBag.SignalRUrl = "http://localhost:2893/SignalR";
}
@section Scripts {
<script src="~/Scripts/jquery.signalR-2.0.2.js"></script> <script src="@String.Concat(@ViewBag.SignalRUrl, "/js")" type="text/javascript"></script> <script src="~/Scripts/tms-Firmware-Upload.js"> </script>
}
|
<input id="fileBrowser" name="fileBrowser" type="file" multiple />
<input id="upload" type="button" class="tms-Button-Inquire" value="Upload" />
<input id="signalRConnId" type="hidden" />
<div id="dropFileZone" name="dropFileZone" class="tms-Div-DropFileZone">
<p class="tms-Label-Laylow">Drag your files
here</p>
</div>
<hr />
<div id="fileDiv" class="tms-Div-Main" style="text-align:left"></div>
<progress id="progress" class="tms-ProgressBar-Upload" style="width:100%" value="0" max="100" />
|
PS. Div : fileDiv is just for output message.
4. jQuery:
■ Ajax for uploading files
//Ajax file to
back-end
function AjaxFiles(data)
{
//initial UI
$('#fileDiv').html("");
$('#fileDiv').append('<li><strong>Start uploading
files ...</strong></li>');
//Upload service url from WebAPI
var url = "http://localhost:2893/api/File/upload?&connId="+connId;
//Send files
$.ajax({
url: url,
data: data,
type: 'POST',
cache: false,
contentType: false,
processData: false,
datatype: 'jsonp'
}).success(
function (res) {
console.log('Upload
Successfully!');
}
).fail(
function (jqxhr, textstatus, err) {
console.log("Upload
failed:" + err);
});
}
|
//Upload Button
Click event
$(function () {
$('#upload').click(function (e) {
var fwFiles = $('#fileBrowser').prop("files");
if (window.FormData != undefined) {
var formData = new FormData();
$.each(fwFiles, function (i, file) {
ParseFile(file);
var filename = file.name;
// Use a regular expression to trim
everything before final dot
var extension =
GetExtension(filename);
console.log(extension);
switch (extension) {
case 'jpg':
default:
formData.append(file.name, fwFiles[i]);
}
});
AjaxFiles(formData);
}
});
});
|
//抑制瀏覽器原有的拖拉操作效果
function
StopDragEvent(evt) {
evt.stopPropagation();
evt.preventDefault();
}
$(function () {
var $dropZone = $("#dropFileZone"); //Drop file div
$dropZone.bind("dragover", function (e) {
StopDragEvent(e);
$(e.target).addClass("tms-Div-Hover");
}).bind("dragleave", function (e) {
StopDragEvent(e);
$(e.target).removeClass("tms-Div-Hover");
}).bind("drop", function (e) {
StopDragEvent(e);
$(e.target).removeClass("tms-Div-Hover");
//上傳檔案
//由dataTransfer.files取得檔案資訊
var files = e.originalEvent.dataTransfer.files;
var fwFiles = $.map(files,
function (f, i) {
//只留下type為image/*者,例如: image/gif, image/jpeg, image/png...
//return
f.type.indexOf("image") == 0 ? f : null;
return f;
});
if (fwFiles.length > 0) {
console.log("上傳檔案數:" +
fwFiles.length);
//FileSelectHandler(e);
//逐一讀入各圖檔,取得DataURI,顯示在網頁上
if (window.FormData != undefined) {
var formData = new FormData();
$.each(fwFiles, function (i, file) {
formData.append(file.name, fwFiles[i]);
});
AjaxFiles(formData);
}
}
});
});
|
■ SignalR Connection and update uploading status with Progressbar.
$(function () {
//UI Setup ----------------------------------------
$('#uploadReport').hide();
//SignalR -----------------------------------------
var signalRUrl = $('#signalRUrl').val();
jQuery.support.cors
= true;
$.connection.hub.url
= signalRUrl;
var myHub =
$.connection.uploaderhub;
$.connection.hub.start();
//Server端傳回上傳進度時,更新檔案狀態
myHub.client.updateProgress = function (name, percentage, message) {
var progress = $('#progress');
progress.attr("value", percentage);
progress.val = message;
///Html encode display name and
message.
var encodedName = $('<div />').text(name).html();
var encodedMsg = $('<div />').text(message).html();
// Add the message to the page.
$('#fileDiv').append('<li><strong>' + encodedName
+ '</strong> ' + encodedMsg + '</li>');
};
});
|
5. Controller:
Skip.
Skip.
6. Reference
HTML5上傳作業進度條-SignalR進階版 (黑大)
HTML5上傳作業進度條-SignalR進階版 (黑大)
沒有留言:
張貼留言